Introducción
Se pretende prender un foco vía bluetooth con arduino y android.Requerimientos de software
- IDE Arduino Studio
- fritzing
- Andiod Studio
Materiales de electrónica y electricidad.
Arduino UNO R3 compatible con IDE Arduino Studio |
![]() |
Protoboard |
![]() |
Modulo relevador Relay 1 canal 5v |
![]() |
Modulo Bluetooth Hc-05 Maestro/esclavo Arduino Pic |
![]() |
Cables Jumpers Dupont m-m h-m h-h |
![]() |
Foco cable #14 y socket |
![]() |

Diagrama

Arduino
Cuando compiles y subas el código en arduino no olvidar quitar los cable RX y TX de hc-06 porque sino te marca un error y no deja subir el código a arduino.
#include <SoftwareSerial.h>
int relay = 2;
SoftwareSerial BT(0,1);
String readdata;
char c;
void setup() {
// put your setup code here, to run once:
BT.begin(9600);
Serial.begin(9600);
pinMode(relay, OUTPUT);
digitalWrite(relay,HIGH);
readdata.reserve(200);
}
void loop() {
// put your main code here, to run repeatedly:
while (BT.available()) { //Check if there is an available byte to read
c = BT.read(); //Conduct a serial read
// readdata += c;
Serial.println(c);
if(c=='a') digitalWrite(relay,LOW);
if(c=='b') digitalWrite(relay,HIGH);
}c="";
}
Andriod Studio
El programa de android lo realice en el api nivel 21 si tu quieres actualizarlo se te queda de tarea y quieres compartir mi correo es kapo1978@hotmail.com mi nombre José Martín Lara.Abrimos nuestro Android Studio y selecionamos New Project selecionamos un Empty Activity y presionamos Next

El nombre de nuestra aplicación BluetoothLightEl nivel de nuestra api: 21 el package name: com.tutosoftware.bluetoothlight y presionamos Finish

Nos vamos al directorio app/manifests Y en el archivo AndroidManifest.xml agregamos los permisos para manejar el bluetooth.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.tutosoftware.bluetoothlight">
<uses-permission android:name="android.permission.BLUETOOTH_SCAN" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.BluetoothLight">
<activity
android:name=".ManoControl"
android:exported="false" />
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_CONNECT" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
</manifest>
Ahora nos vamos al directorio app/res/layout y en el archivo activity_main.xml agregamos los siguientes elementos.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:layout_width="match_parent"
tools:orientation="vertical">
<TextView android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:id="@+id/texto"
android:text="Dispositivos Emparejados"/>
<Button android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:id="@+id/boton"
android:text="Emparejar dispositivos"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"/>
<ListView android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:id="@+id/listView"
android:layout_below="@+id/texto"
android:layout_above="@+id/boton"
android:layout_centerHorizontal="true"/>
</RelativeLayout>
En la clase MainActivity.java escribimos lo siguiente:
package com.tutosoftware.bluetoothlight;
import androidx.appcompat.app.AppCompatActivity;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Set;
public class MainActivity extends AppCompatActivity {
Button btnEmparejar;
ListView listaDispositivos;
//Bluetooth
private BluetoothAdapter myBluetooth = null;
private Set<BluetoothDevice> pairedDevices;
public static String EXTRA_ADDRESS = "device_address";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnEmparejar = (Button) findViewById(R.id.boton);
listaDispositivos = (ListView) findViewById(R.id.listView);
//if the device has bluetooth
myBluetooth = BluetoothAdapter.getDefaultAdapter();
if (myBluetooth == null) {
//Show a mensag. that the device has no bluetooth adapter
Toast.makeText(getApplicationContext(), "El dispositivo bluetooht no esta disponible", Toast.LENGTH_LONG).show();
//finish apk
finish();
} else if (!myBluetooth.isEnabled()) {
//Ask to the user turn the bluetooth on
Intent turnBTon = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(turnBTon, 1);
}
btnEmparejar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
pairedDevicesList();
}
});
}
private void pairedDevicesList()
{
pairedDevices = myBluetooth.getBondedDevices();
ArrayList list = new ArrayList();
if (pairedDevices.size()>0)
{
for(BluetoothDevice bt : pairedDevices)
{
list.add(bt.getName() + "\n" + bt.getAddress()); //Get the device's name and the address
}
}
else
{
Toast.makeText(getApplicationContext(), "No Paired Bluetooth Devices Found.", Toast.LENGTH_LONG).show();
}
final ArrayAdapter adapter = new ArrayAdapter(this,android.R.layout.simple_list_item_1, list);
listaDispositivos.setAdapter(adapter);
listaDispositivos.setOnItemClickListener(myListClickListener); //Method called when the device from the list is clicked
}
private AdapterView.OnItemClickListener myListClickListener = new AdapterView.OnItemClickListener()
{
public void onItemClick (AdapterView<?> av, View v, int arg2, long arg3)
{
// Get the device MAC address, the last 17 chars in the View
String info = ((TextView) v).getText().toString();
String address = info.substring(info.length() - 17);
// Make an intent to start next activity.
Intent i = new Intent(MainActivity.this, ManoControl.class);
//Change the activity.
i.putExtra(EXTRA_ADDRESS, address); //this will be received at ledControl (class) Activity
startActivity(i);
}
};
}
Ahora creamos una Activity selecionamos New->Activity->Empty Activity y la nombramos ManoControl

Ahora nos vamos al directorio app/res/layout y en el archivo activity_mano_control.xml agregamos los siguientes elementos.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".ManoControl">
<Button
android:id="@+id/uno"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="0dp"
android:layout_marginLeft="100dp"
android:text="Apagar" />
<Button
android:id="@+id/dos"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:layout_marginLeft="100dp"
android:text="Encender" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Desconectar"
android:id="@+id/des"
android:layout_marginTop="500dp"
android:layout_marginLeft="100dp"
/>
</RelativeLayout>
Por último nos vamos a la clase ManoControl.java
package com.tutosoftware.bluetoothlight;
import androidx.appcompat.app.AppCompatActivity;
import android.app.ProgressDialog;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.content.Intent;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import java.io.IOException;
import java.util.UUID;
public class ManoControl extends AppCompatActivity {
Button apagar,prender,desconectar;
String address = null;
BluetoothAdapter myBluetooth = null;
BluetoothSocket btSocket = null;
private boolean isBtConnected = false;
//SPP UUID. Look for it
static final UUID myUUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private ProgressDialog progress;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_mano_control);
Intent newint = getIntent();
address = newint.getStringExtra(MainActivity.EXTRA_ADDRESS);
prender = (Button) findViewById(R.id.uno);
apagar = (Button) findViewById(R.id.dos);
desconectar = (Button) findViewById(R.id.des);
new ConnectBT().execute();
apagar.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View v)
{
mandarComando("a"); //method to turn on
}
});
prender.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View v)
{
mandarComando("b"); //method to turn on
}
});
desconectar.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View v)
{
Disconnect(); //close connection
}
});
}
private void Disconnect()
{
if (btSocket!=null) //If the btSocket is busy
{
try
{
btSocket.close(); //close connection
}
catch (IOException e)
{ msg("Error");}
}
finish(); //return to the first layout
}
private void mandarComando(String comando)
{
if (btSocket!=null)
{
try
{
btSocket.getOutputStream().write(comando.getBytes());
}
catch (IOException e)
{
msg("Error");
}
}
}
private void msg(String s)
{
Toast.makeText(getApplicationContext(),s, Toast.LENGTH_LONG).show();
}
private class ConnectBT extends AsyncTask<Void, Void, Void> // UI thread
{
private boolean ConnectSuccess = true; //if it's here, it's almost connected
@Override
protected void onPreExecute()
{
progress = ProgressDialog.show(ManoControl.this, "Connecting...", "Please wait!!!"); //show a progress dialog
}
@Override
protected Void doInBackground(Void... devices) //while the progress dialog is shown, the connection is done in background
{
try
{
if (btSocket == null || !isBtConnected)
{
myBluetooth = BluetoothAdapter.getDefaultAdapter();//get the mobile bluetooth device
BluetoothDevice dispositivo = myBluetooth.getRemoteDevice(address);//connects to the device's address and checks if it's available
btSocket = dispositivo.createInsecureRfcommSocketToServiceRecord(myUUID);//create a RFCOMM (SPP) connection
BluetoothAdapter.getDefaultAdapter().cancelDiscovery();
btSocket.connect();//start connection
}
}
catch (IOException e)
{
ConnectSuccess = false;//if the try failed, you can check the exception here
}
return null;
}
@Override
protected void onPostExecute(Void result) //after the doInBackground, it checks if everything went fine
{
super.onPostExecute(result);
if (!ConnectSuccess)
{
msg("Connection Failed. Is it a SPP Bluetooth? Try again.");
finish();
}
else
{
msg("Connected.");
isBtConnected = true;
}
progress.dismiss();
}
}
}
apk
Les dejo el apk por si se les dificulta programar en andriod una vez que instalen el apk y lo prueban, si no les detecta el hc-o6 Se van a ajustes y en la sección de bluetooth lo emparejan primero y les va a pedir una contraseña por lo regular es 1234 una vez instalada en ajustes cierran la aplicación y la vuelven a abrir y pueden observar que ya detecta el hc-06 y mi caso dice hc-05.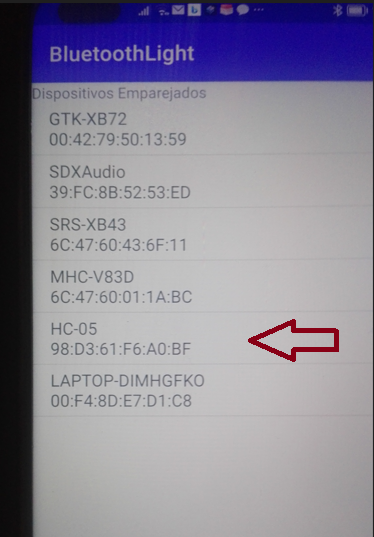