Modulo de ventas online
Si quisieras comercializar en la web tus productos que tienes en tu inventario
logico que necesitas un modulo de ventas-online este modulo simula la venta de tus
productos en la web asi que comenzemos.
Lo primero es que vamos realizar es crear las tablas pedido,ventadetalleonline,ventaonline estas las encuentras al comienzo
del tutorial.
Nos vamos al proyecto productmarket-core y en el paquete com.tutosoftware.productmarket.entity vamos
a crear las entidades y los modelos de sistema comenzemos con la entidad o clase Pedido.java escribimos el siguiente código:
package com.tutosoftware.productmarket.entity;
import java.io.Serializable;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="pedido")
public class Pedido implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private long idPedido;
@Column(name="llave")
private String llave;
@Column(name="nombre")
private String nombre;
@Column(name="apellidopat")
private String apellidoPaterno;
@Column(name="apellidomat")
private String apellidoMaterno;
@Column(name="calle")
private String calle;
@Column(name="entrecalles")
private String entrecalles;
@Column(name="colonia")
private String colonia;
@Column(name="municipio")
private String municipio;
@Column(name="estado")
private String estado;
@Column(name="pais")
private String pais;
@Column(name="codigopostal")
private String codigoPostal;
@Column(name="telefono")
private String telefono;
@Column(name="email")
private String email;
@Column(name="fecha")
private Date fecha;
public long getIdPedido() {
return idPedido;
}
public void setIdPedido(long idPedido) {
this.idPedido = idPedido;
}
public String getLlave() {
return llave;
}
public void setLlave(String llave) {
this.llave = llave;
}
public String getNombre() {
return nombre;
}
public void setNombre(String nombre) {
this.nombre = nombre;
}
public String getApellidoPaterno() {
return apellidoPaterno;
}
public void setApellidoPaterno(String apellidoPaterno) {
this.apellidoPaterno = apellidoPaterno;
}
public String getApellidoMaterno() {
return apellidoMaterno;
}
public void setApellidoMaterno(String apellidoMaterno) {
this.apellidoMaterno = apellidoMaterno;
}
public String getCalle() {
return calle;
}
public void setCalle(String calle) {
this.calle = calle;
}
public String getEntrecalles() {
return entrecalles;
}
public void setEntrecalles(String entrecalles) {
this.entrecalles = entrecalles;
}
public String getColonia() {
return colonia;
}
public void setColonia(String colonia) {
this.colonia = colonia;
}
public String getMunicipio() {
return municipio;
}
public void setMunicipio(String municipio) {
this.municipio = municipio;
}
public String getEstado() {
return estado;
}
public void setEstado(String estado) {
this.estado = estado;
}
public String getPais() {
return pais;
}
public void setPais(String pais) {
this.pais = pais;
}
public String getCodigoPostal() {
return codigoPostal;
}
public void setCodigoPostal(String codigoPostal) {
this.codigoPostal = codigoPostal;
}
public String getTelefono() {
return telefono;
}
public void setTelefono(String telefono) {
this.telefono = telefono;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Date getFecha() {
return fecha;
}
public void setFecha(Date fecha) {
this.fecha = fecha;
}
}
La siguiente clase que vamos a agregar es VentaDetalleOnline.java Esta
nos va a servir para agregar todas las ventas que realizemos a nuestro reporteador.
package com.tutosoftware.productmarket.entity;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Id;
@Entity
@Table(name="ventadetalleonline")
public class VentaDetalleOnline implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private long idPedido;
@Column(name="cantidad")
private Integer cantidad;
@Column(name="idproducto")
private long idProducto;
@Column(name="costoProducto")
private Double costoProducto;
public long getIdPedido() {
return idPedido;
}
public void setIdPedido(long idPedido) {
this.idPedido = idPedido;
}
public Integer getCantidad() {
return cantidad;
}
public void setCantidad(Integer cantidad) {
this.cantidad = cantidad;
}
public long getIdProducto() {
return idProducto;
}
public void setIdProducto(long idProducto) {
this.idProducto = idProducto;
}
public Double getCostoProducto() {
return costoProducto;
}
public void setCostoProducto(Double costoProducto) {
this.costoProducto = costoProducto;
}
}
Ahora vamos registrar las ventas totales por pedido creamos la clase VentaOnline.java y escribimos:
package com.tutosoftware.productmarket.entity;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="ventaonline")
public class VentaOnline implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private long idpedido;
@Column(name="total")
private Double total;
public long getIdpedido() {
return idpedido;
}
public void setIdpedido(long idpedido) {
this.idpedido = idpedido;
}
public Double getTotal() {
return total;
}
public void setTotal(Double total) {
this.total = total;
}
}
Ahora vamos con los modelos estos tambien los vamos a agregar en el paquete
com.tutosoftware.productmarket.entity aunque puedes separarlo, nombrar uno llamado models.
pero como ya no tengo tiempo lo vamos a dejar en ese paquete creamos la clase
Item.java es nos sirve para agregar los productos al carrito de compra con sesiones.
package com.tutosoftware.productmarket.entity;
import com.tutosoftware.productmarket.entity.Producto;
public class Item implements Serializable{
private static final long serialVersionUID = 1L;
private Producto producto = new Producto();
private int cantidad;
public Producto getProducto() {
return producto;
}
public void setProducto(Producto producto) {
this.producto = producto;
}
public int getCantidad() {
return cantidad;
}
public void setCantidad(int cantidad) {
this.cantidad = cantidad;
}
public Item(Producto producto, int cantidad) {
super();
this.producto = producto;
this.cantidad = cantidad;
}
public Item() {
super();
}
}
La siguiente clase seria Orden.java para agregar nuestro pedido.
package com.tutosoftware.productmarket.entity;
import org.hibernate.validator.constraints.Email;
import org.hibernate.validator.constraints.NotBlank;
public class Orden {
@NotBlank
private String nombre;
@NotBlank
private String apellidoPaterno;
private String apellidoMaterno;
@NotBlank
private String calle;
private String entrecalles;
@NotBlank
private String colonia;
@NotBlank
private String municipio;
@NotBlank
private String estado;
@NotBlank
private String pais;
@NotBlank
private String codigoPostal;
@NotBlank
private String telefono;
@Email
@NotBlank
private String email;
public String getNombre() {
return nombre;
}
public void setNombre(String nombre) {
this.nombre = nombre;
}
public String getApellidoPaterno() {
return apellidoPaterno;
}
public void setApellidoPaterno(String apellidoPaterno) {
this.apellidoPaterno = apellidoPaterno;
}
public String getApellidoMaterno() {
return apellidoMaterno;
}
public void setApellidoMaterno(String apellidoMaterno) {
this.apellidoMaterno = apellidoMaterno;
}
public String getCalle() {
return calle;
}
public void setCalle(String calle) {
this.calle = calle;
}
public String getEntrecalles() {
return entrecalles;
}
public void setEntrecalles(String entrecalles) {
this.entrecalles = entrecalles;
}
public String getColonia() {
return colonia;
}
public void setColonia(String colonia) {
this.colonia = colonia;
}
public String getMunicipio() {
return municipio;
}
public void setMunicipio(String municipio) {
this.municipio = municipio;
}
public String getEstado() {
return estado;
}
public void setEstado(String estado) {
this.estado = estado;
}
public String getPais() {
return pais;
}
public void setPais(String pais) {
this.pais = pais;
}
public String getCodigoPostal() {
return codigoPostal;
}
public void setCodigoPostal(String codigoPostal) {
this.codigoPostal = codigoPostal;
}
public String getTelefono() {
return telefono;
}
public void setTelefono(String telefono) {
this.telefono = telefono;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Lo que sigue a continuación es movernos al paquete com.tutosoftware.productmarket.dao y creamos
la interface VentasOnlineDAO.java
package com.tutosoftware.productmarket.dao;
import java.util.List;
import com.tutosoftware.productmarket.entity.Pedido;
import com.tutosoftware.productmarket.entity.Producto;
import com.tutosoftware.productmarket.entity.VentaDetalleOnline;
import com.tutosoftware.productmarket.entity.VentaOnline;
public interface VentasOnlineDAO {
public List<Producto> obtenerProductos(String nombreProducto);
public long agregarPedido(Pedido pedido);
public Pedido obtenerPedido(String llavePedido);
public long agregarVentaDetalleOnline(VentaDetalleOnline ventaDetalleOnline);
public long agregarVentaOnline(VentaOnline ventaOnline);
}
Para crear la implementación nos vamos al paquete com.tutosoftware.productmarket.dao.impl y
creamos la clase VentasOnlineDAOImpl.java
package com.tutosoftware.productmarket.dao.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import com.tutosoftware.productmarket.dao.VentasOnlineDAO;
import com.tutosoftware.productmarket.entity.Pedido;
import com.tutosoftware.productmarket.entity.Producto;
import com.tutosoftware.productmarket.entity.VentaDetalleOnline;
import com.tutosoftware.productmarket.entity.VentaOnline;
import com.tutosoftware.productmarket.util.HibernateUtil;
@Repository
public class VentasOnlineDAOImpl implements VentasOnlineDAO {
@Autowired
private HibernateUtil hibernateUtil;
VentasOnlineDAOImpl(){
}
@Override
public List<Producto> obtenerProductos(String nombreProducto) {
String query = "FROM Producto p WHERE p.nombreProducto like '%"+ nombreProducto +"%' and p.cantidad > 0 ";
return hibernateUtil.fetchAll(query);
}
@Override
public long agregarPedido(Pedido pedido) {
// TODO Auto-generated method stub
return (Long) hibernateUtil.create(pedido);
}
@Override
public Pedido obtenerPedido(String llavePedido) {
String hql ="from Pedido as p where p.llave = '"+llavePedido+"'";
Pedido pedidoResult = hibernateUtil.fetchByUniqueResult(hql);
return pedidoResult;
}
@Override
public long agregarVentaDetalleOnline(VentaDetalleOnline ventaDetalleOnline) {
// TODO Auto-generated method stub
return (Long) hibernateUtil.create(ventaDetalleOnline);
}
@Override
public long agregarVentaOnline(VentaOnline ventaOnline) {
// TODO Auto-generated method stub
return (Long) hibernateUtil.create(ventaOnline);
}
}
Lo que sigue a continuación es nuestro servicio vamos al paquete com.tutosoftware.productmarket.service
y creamos la interface VentasOnlineService.java
package com.tutosoftware.productmarket.service;
import java.util.List;
import com.tutosoftware.productmarket.entity.Pedido;
import com.tutosoftware.productmarket.entity.Producto;
import com.tutosoftware.productmarket.entity.VentaDetalleOnline;
import com.tutosoftware.productmarket.entity.VentaOnline;
public interface VentasOnlineService {
public List<Producto> obtenerProductos(String nombreProducto);
public long agregarPedido(Pedido pedido);
public Pedido obtenerPedido(String llavePedido);
public long agregarVentaDetalleOnline(VentaDetalleOnline ventaDetalleOnline);
public long agregarVentaOnline(VentaOnline ventaOnline);
}
Para la implementación nos vamos al paquete com.tutosoftware.productmarket.service.impl y escribimos
la clase llamada VentasOnlineServiceImpl
package com.tutosoftware.productmarket.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.tutosoftware.productmarket.dao.VentasOnlineDAO;
import com.tutosoftware.productmarket.entity.Pedido;
import com.tutosoftware.productmarket.entity.Producto;
import com.tutosoftware.productmarket.entity.VentaDetalleOnline;
import com.tutosoftware.productmarket.entity.VentaOnline;
import com.tutosoftware.productmarket.service.VentasOnlineService;
@Service
@Transactional
public class VentasOnlineServiceImpl implements VentasOnlineService {
@Autowired
private VentasOnlineDAO ventasOnlineDAO;
public VentasOnlineServiceImpl(){
}
@Override
public List<Producto> obtenerProductos(String nombreProducto) {
// TODO Auto-generated method stub
return ventasOnlineDAO.obtenerProductos(nombreProducto);
}
@Override
public long agregarPedido(Pedido pedido) {
// TODO Auto-generated method stub
return ventasOnlineDAO.agregarPedido(pedido);
}
@Override
public Pedido obtenerPedido(String llavePedido) {
// TODO Auto-generated method stub
return ventasOnlineDAO.obtenerPedido(llavePedido);
}
@Override
public long agregarVentaDetalleOnline(VentaDetalleOnline ventaDetalleOnline) {
// TODO Auto-generated method stub
return ventasOnlineDAO.agregarVentaDetalleOnline(ventaDetalleOnline);
}
@Override
public long agregarVentaOnline(VentaOnline ventaOnline) {
// TODO Auto-generated method stub
return ventasOnlineDAO.agregarVentaOnline(ventaOnline);
}
}
Ahora nos vamos al proyecto productmarket-ventaonline y el archivo pom.xml
escribimos lo siguiente:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.tutosoftware.productmarket</groupId>
<artifactId>productmarket</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>productmarket-ventaonline</artifactId>
<packaging>war</packaging>
<build>
<finalName>productmarketonline</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.3</version>
<configuration>
<webXml>src/main/webapp/WEB-INF/web.xml</webXml>
<webResources>
<resource>
<!-- this is relative to the pom.xml directory -->
<directory>src/main/webapp/WEB-INF</directory>
<targetPath>WEB-INF</targetPath>
</resource>
</webResources>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>com.tutosoftware.productmarket</groupId>
<artifactId>productmarket-core</artifactId>
</dependency>
<!-- Spring 4 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
</dependency>
<!-- Hibernate dependencies -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-c3p0</artifactId>
</dependency>
<!-- mysql dependencie -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- Servlet and JSP -->
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>jsp-api</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
</dependencies>
</project>
Ahora nos vamos a src/main/webapp/WEB-INF y creamos el arhivo mvc-dispatcher-servlet.xml y
escribimos el siguiente código:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!-- Specifying base package of the Components like Controller, Service,
DAO -->
<context:component-scan base-package="com.tutosoftware.productmarket" />
<!-- Getting Database properties -->
<context:property-placeholder location="classpath:application.properties" />
<!-- Specifying the Resource location to load JS, CSS, Images etc -->
<mvc:annotation-driven />
<mvc:resources mapping="/resources/**" location="/resources/" />
<mvc:resources mapping="/css/**" location="/css/" />
<mvc:resources mapping="/fonts/**" location="/fonts/" />
<mvc:resources mapping="/js/**" location="/js/" />
<!-- View Resolver -->
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
<bean id="messageSource"
class="org.springframework.context.support.ResourceBundleMessageSource">
<property name="basename" value="mensaje"/>
</bean>
<!-- DataSource -->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"
destroy-method="close">
<property name="driverClass" value="${database.driverClass}" />
<property name="jdbcUrl" value="${database.url}" />
<property name="user" value="${database.username}" />
<property name="password" value="${database.password}" />
<property name="acquireIncrement" value="${connection.acquireIncrement}" />
<property name="minPoolSize" value="${connection.minPoolSize}" />
<property name="maxPoolSize" value="${connection.maxPoolSize}" />
<property name="maxIdleTime" value="${connection.maxIdleTime}" />
</bean>
<!-- Hibernate SessionFactory -->
<bean id="sessionFactory"
class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">${hibernate.dialect}</prop>
<prop key="hibernate.hbm2ddl.auto">${hibernate.hbm2ddl.auto}</prop>
<prop key="hibernate.format_sql">${hibernate.format_sql}</prop>
<prop key="hibernate.show_sql">${hibernate.show_sql}</prop>
</props>
</property>
<property name="packagesToScan" value="com.tutosoftware.productmarket.entity"></property>
</bean>
<!-- Transaction -->
<bean id="transactionManager"
class="org.springframework.orm.hibernate5.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
<tx:annotation-driven transaction-manager="transactionManager" />
</beans>
Ahora nos vamos a src/main/resources y creamos el archivo application.resources no olvidar
meter tu usuario y password de tu base datos:
database.driverClass=com.mysql.jdbc.Driver
database.url=jdbc:mysql://localhost:3306/productmarketdb
database.username=usuario
database.password=password
#Hibernate related properties
hibernate.dialect=org.hibernate.dialect.MySQL5Dialect
hibernate.hbm2ddl.auto=update
hibernate.show_sql=true
hibernate.format_sql=true
#Connection pool related properties
connection.acquireIncrement=2
connection.minPoolSize=20
connection.maxPoolSize=50
connection.maxIdleTime=600
En ese mismo directorio de src/main/resourcesy creamos el archivo mensaje.properties esto te sirve
para validaciones e internacionalización
NotBlank=Este campo no puede estar en blanco
Email=La direcci\u00f3n de correo no es v\u00e1lida
Lo que sigue es irnos a src/main/java y creamos el paquete com.tutosoftware.productmarket.controller y
en este creamos la clase controlador VentasOnlineController.java
package com.tutosoftware.productmarket.controller;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.ui.ModelMap;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import com.tutosoftware.productmarket.entity.Item;
import com.tutosoftware.productmarket.entity.Orden;
import com.tutosoftware.productmarket.entity.Pedido;
import com.tutosoftware.productmarket.entity.Producto;
import com.tutosoftware.productmarket.entity.VentaDetalleOnline;
import com.tutosoftware.productmarket.entity.VentaOnline;
import com.tutosoftware.productmarket.service.ProductoService;
import com.tutosoftware.productmarket.service.VentasOnlineService;
@Controller
public class VentasOnlineController {
@Autowired
private VentasOnlineService ventasOnlineService;
@Autowired
private ProductoService productoService;
public VentasOnlineController(){
}
@RequestMapping(value = {"/buscarProductos", "/"})
public ModelAndView buscarProducto() {
return new ModelAndView("buscar");
}
@RequestMapping("/buscarProducto")
public ModelAndView mostrarProducto(@RequestParam("nombreProducto") String nombreProducto,ModelMap model) {
if(nombreProducto.trim().length() != 0 ){
ModelAndView modelo = new ModelAndView("mostrarProducto");
List<Producto> productoList = ventasOnlineService.obtenerProductos(nombreProducto);
modelo.addObject("productoList",productoList);
return modelo;
}else{
model.put("vacio","Escribe el nombre del producto");
return new ModelAndView("buscar");
}
}
@SuppressWarnings (value="unchecked")
@RequestMapping("/agregarCompra")
public ModelAndView agregarCarritoCompra(@RequestParam long id,HttpSession session) {
Producto producto = null;
producto = productoService.obtenerProducto(id);
if(session.getAttribute("cart") == null){
List<Item> cart = new ArrayList<Item>();
cart.add(new Item(producto,1));
session.setAttribute("cart",cart);
}else{
List<Item> cart = (List<Item>)session.getAttribute("cart");
int index = siExisteProductoCompra(id,session);
if(index == -1)
cart.add(new Item(producto,1));
else{
int cantidad = cart.get(index).getCantidad()+1;
cart.get(index).setCantidad(cantidad);
}
session.setAttribute("cart",cart);
}
return new ModelAndView("informacionCompra");
}
@RequestMapping("/verCompra")
public ModelAndView verCarritoCompra() {
return new ModelAndView("informacionCompra");
}
@SuppressWarnings (value="unchecked")
private int siExisteProductoCompra(long id,HttpSession session){
List<Item> cart = (List<Item>)session.getAttribute("cart");
for(int i=0;i < cart.size();i++)
if(cart.get(i).getProducto().getIdProducto() == id)
return i;
return -1;
}
@SuppressWarnings (value="unchecked")
@RequestMapping("/borrarProducto")
public ModelAndView eliminarCarritoCompra(@RequestParam long id,HttpSession session) {
List<Item> cart = (List<Item>)session.getAttribute("cart");
int index = siExisteProductoCompra(id,session);
cart.remove(index);
session.setAttribute("cart",cart);
return new ModelAndView("informacionCompra");
}
@RequestMapping("/comprar")
public ModelAndView pedido(@ModelAttribute Orden orden){
//session.removeAttribute("cart");
return new ModelAndView("pedido");
}
@RequestMapping("/registrar")
public ModelAndView registrarPedido(@Valid Orden orden, BindingResult result){
ModelAndView model = new ModelAndView();
model.addObject("orden", orden);
model.setViewName(result.hasErrors() ? "pedido" : "comprar");
//session.removeAttribute("cart");
return model;
}
@RequestMapping("/cancelarCompra")
public ModelAndView cancelar(HttpSession session){
session.removeAttribute("cart");
return new ModelAndView("buscar");
}
@RequestMapping("/pagar")
public ModelAndView pagarProductos(@ModelAttribute Orden orden,HttpSession session){
String llavePedido = UUID.randomUUID().toString();
agregarPedidoBD(orden,llavePedido);
Pedido pedido = ventasOnlineService.obtenerPedido(llavePedido);
//System.out.println(pedido.getIdPedido());
agregarVentaDetalleBD(pedido.getIdPedido(),session);
session.removeAttribute("cart");
return new ModelAndView("buscar");
}
@RequestMapping("/imagenProducto")
public void imagenProducto(HttpServletRequest request, HttpServletResponse response, Model model,
@RequestParam long id)throws IOException{
Producto producto = null;
producto = productoService.obtenerProducto(id);
response.setContentType("image/jpeg, image/jpg, image/png, image/gif");
response.getOutputStream().write(producto.getImagen());
response.getOutputStream().close();
}
private void agregarPedidoBD(Orden orden,String llavePedido){
Pedido pedido = new Pedido();
pedido.setLlave(llavePedido);
pedido.setNombre(orden.getNombre());
pedido.setApellidoPaterno(orden.getApellidoPaterno());
pedido.setApellidoMaterno(orden.getApellidoMaterno());
pedido.setCalle(orden.getCalle());
pedido.setEntrecalles(orden.getEntrecalles());
pedido.setColonia(orden.getColonia());
pedido.setMunicipio(orden.getMunicipio());
pedido.setEstado(orden.getEstado());
pedido.setPais(orden.getPais());
pedido.setCodigoPostal(orden.getCodigoPostal());
pedido.setTelefono(orden.getTelefono());
pedido.setEmail(orden.getEmail());
pedido.setFecha(new Date());
ventasOnlineService.agregarPedido(pedido);
//System.out.println("***************Probando metodo**************************");
//System.out.println(llavePedido);
//System.out.println(orden.getNombre());
}
@SuppressWarnings (value="unchecked")
private void agregarVentaDetalleBD(long idPedido,HttpSession session){
VentaDetalleOnline ventaDetalleOnline = new VentaDetalleOnline();
List<Item> cart = (List<Item>)session.getAttribute("cart");
Producto productoActualizarInventario = new Producto();
VentaOnline ventasOnline = new VentaOnline();
double totalVentas=0;
for(int i=0;i < cart.size();i++){
ventaDetalleOnline.setIdPedido(idPedido);
ventaDetalleOnline.setCantidad(cart.get(i).getCantidad());
ventaDetalleOnline.setIdProducto(cart.get(i).getProducto().getIdProducto());
ventaDetalleOnline.setCostoProducto(cart.get(i).getProducto().getPrecio() * cart.get(i).getCantidad());
ventasOnlineService.agregarVentaDetalleOnline(ventaDetalleOnline);
productoActualizarInventario = cart.get(i).getProducto();
productoActualizarInventario.setCantidad(cart.get(i).getProducto().getCantidad()-cart.get(i).getCantidad());
productoService.actualizarProducto(productoActualizarInventario);
totalVentas += cart.get(i).getProducto().getPrecio() * cart.get(i).getCantidad();
}
ventasOnline.setIdpedido(idPedido);
ventasOnline.setTotal(totalVentas);
ventasOnlineService.agregarVentaOnline(ventasOnline);
}
}
En src/main/webapp/WEB-INF creamos la carpeta views la primera vista que
vamos crear la vamos a nombrar buscar.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="http://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="css/style.css" />
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection"/>
<script type="text/javascript" src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<title>Shop Producto market</title>
</head>
<body>
<div class="container">
<form action="buscarProducto" >
<table>
<tr>
<td>
<div class="input-field col s5">
<input type='text' name='nombreProducto' id='nombreProducto'/>
<label for="nombreProducto">BuscarProducto</label>
</div>
</td>
<td>
<button class="btn waves-effect waves-light" type="submit" name="action">buscar
<i class="material-icons right">send</i>
</button>
</td>
</tr>
</table>
<p><span class="red accent-4">${vacio}</span></p>
</form>
<c:set var="t" value="0"></c:set>
<c:forEach var="c" items="${sessionScope.cart}">
<c:set var="t" value="${t + c.producto.precio * c.cantidad}"></c:set>
</c:forEach>
<h4>Total Compra: ${t} </h4> <a href="verCompra" class="waves-effect waves-light btn">Detalle Compra</a>
<a href="comprar" class="waves-effect waves-light btn">Comprar</a>
<a href="cancelarCompra" class="waves-effect waves-light btn">Cancelar Compra</a>
<div class="carousel carousel-slider center" data-indicators="true">
<div class="carousel-item red white-text" href="#one!">
<h2>First Panel</h2>
<p class="white-text">This is your first panel</p>
</div>
<div class="carousel-item amber white-text" href="#two!">
<h2>Second Panel</h2>
<p class="white-text">This is your second panel</p>
</div>
<div class="carousel-item green white-text" href="#three!">
<h2>Third Panel</h2>
<p class="white-text">This is your third panel</p>
</div>
<div class="carousel-item blue white-text" href="#four!">
<h2>Fourth Panel</h2>
<p class="white-text">This is your fourth panel</p>
</div>
</div>
</div>
<script>
$('.carousel.carousel-slider').carousel({fullWidth: true});
</script>
</body>
</html>
La vista que sigue es mostrarProducto.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="http://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="css/style.css" />
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection"/>
<script type="text/javascript" src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<title>Shop Producto market</title>
</head>
<body>
<div class="container">
<form action="buscarProducto" >
<table>
<tr>
<td>
<div class="input-field col s5">
<input type='text' name='nombreProducto' id='nombreProducto'/>
<label for="nombreProducto">BuscarProducto</label>
</div>
</td>
<td>
<button class="btn waves-effect waves-light" type="submit" name="action">buscar
<i class="material-icons right">send</i>
</button>
</td>
</tr>
</table>
</form>
<c:set var="t" value="0"></c:set>
<c:forEach var="c" items="${sessionScope.cart}">
<c:set var="t" value="${t + c.producto.precio * c.cantidad}"></c:set>
</c:forEach>
<h4>Total Compra:${t}</h4>
<a href="verCompra" class="waves-effect waves-light btn">Detalle Compra</a>
<a href="comprar" class="waves-effect waves-light btn">Comprar</a>
<a href="cancelarCompra" class="waves-effect waves-light btn">Cancelar Compra</a>
<c:if test="${empty productoList}">
No hay productos busca otra ocurrencia
</c:if>
<c:if test="${not empty productoList}">
<div class="paging"></div>
<div class="row" >
<c:forEach items="${productoList}" var="pro">
<div class="element">
<div class="col s12 m7">
<h2 class="header"><c:out value="${pro.nombreProducto}"/></h2>
<div class="card horizontal">
<div class="card-image">
<img src="${pageContext.request.contextPath}/imagenProducto?id=${pro.idProducto}" alt="imagen no disponible" />
</div>
<div class="card-stacked">
<div class="card-content">
<table>
<tr>
<td>
<ul class="collection">
<li class="collection-item">Descripcion: </li>
<li class="collection-item">Cantidad disponible:</li>
<li class="collection-item">Precio:</li>
</ul>
</td>
<td>
<ul class="collection">
<li class="collection-item"><c:out value="${pro.descripcion}"/> </li>
<li class="collection-item"><c:out value="${pro.cantidad}"/> </li>
<li class="collection-item">$<c:out value="${pro.precio}"/></li>
</ul>
</td>
</tr>
</table>
</div>
<div class="card-action">
<a href="agregarCompra?id=<c:out value='${pro.idProducto}'/>"
class="waves-effect waves-light btn">Agregar</a>
</div>
</div>
</div>
</div>
</div>
</c:forEach>
</div>
<div class="paging"></div>
</c:if>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.1/jquery.min.js"></script>
<script src="js/jquery.ba-hashchange.js"></script>
<script src="js/jquery.paging.min.js"></script>
<script>
/*
* Slicing the content with two pagers and using the URL Hash event
*/
(function() {
var prev = {
start: 0,
stop: 0
};
var content = $('.element');
var Paging = $(".paging").paging(content.length, {
onSelect: function() {
var data = this.slice;
content.slice(prev[0], prev[1]).css('display', 'none');
content.slice(data[0], data[1]).fadeIn("slow");
prev = data;
return true; // locate!
},
onFormat: function(type) {
switch (type) {
case 'block':
if (!this.active)
return '<span class="disabled">' + this.value + '</span>';
else if (this.value != this.page)
return '<em><a href="#' + this.value + '">' + this.value + '</a></em>';
return '<span class="current">' + this.value + '</span>';
case 'right':
case 'left':
if (!this.active) {
return '';
}
return '<a href="#' + this.value + '">' + this.value + '</a>';
case 'next':
if (this.active) {
return '<a href="#' + this.value + '" class="next">Next »</a>';
}
return '<span class="disabled">Next »</span>';
case 'prev':
if (this.active) {
return '<a href="#' + this.value + '" class="prev">« Previous</a>';
}
return '<span class="disabled">« Previous</span>';
case 'first':
if (this.active) {
return '<a href="#' + this.value + '" class="first">|<</a>';
}
return '<span class="disabled">|<</span>';
case 'last':
if (this.active) {
return '<a href="#' + this.value + '" class="prev">>|</a>';
}
return '<span class="disabled">>|</span>';
case 'fill':
if (this.active) {
return "...";
}
}
return ""; // return nothing for missing branches
},
format: '[< ncnnn! >]',
perpage: 3,
lapping: 0,
page: null // we await hashchange() event
});
$(window).hashchange(function() {
if (window.location.hash)
Paging.setPage(window.location.hash.substr(1));
else
Paging.setPage(1); // we dropped "page" support and need to run it by hand
});
$(window).hashchange();
})();
</script>
</body>
</html>
La que sigue es informacionCompra.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="http://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="css/style.css" />
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection"/>
<script type="text/javascript" src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<title>Información de compra</title>
</head>
<body>
<div class="container">
<table class="bordered">
<thead>
<tr>
<th>Eliminar</th>
<th>Cantidad</th>
<th>Nombre Producto</th>
<th>Precio</th>
<th>Sub total</th>
</tr>
</thead>
<tbody>
<c:set var="t" value="0"></c:set>
<c:forEach var="c" items="${sessionScope.cart}">
<c:set var="t" value="${t + c.producto.precio * c.cantidad}"></c:set>
<tr>
<td><a href="borrarProducto?id=${c.producto.idProducto}" class="waves-effect waves-light btn">BorrarProducto</a></td>
<td>${c.cantidad}</td>
<td>${c.producto.nombreProducto}</td>
<td>${c.producto.precio}</td>
<td>${c.producto.precio * c.cantidad}</td>
</tr>
</c:forEach>
<tr>
<td colspan="4">Total</td>
<td>${t}</td>
</tr>
</tbody>
</table>
<br>
<a href="buscarProductos" class="waves-effect waves-light btn">Continuar comprando</a>
<a href="comprar" class="waves-effect waves-light btn">Comprar</a>
<a href="cancelarCompra" class="waves-effect waves-light btn">Cancelar Compra</a>
</div>
</body>
</html>
Ahora creamos la interfaz gráfica pedido.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="http://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="css/style.css" />
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection"/>
<script type="text/javascript" src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<title>Registra tu pedido</title>
<style type="text/css">
.input-error { margin-bottom: 6px !important; }
.text-error { font-size: 0.8em !important; }
</style>
</head>
<body>
<div class="container">
<h3>Ingresa tu pedido</h3>
<c:set var="t" value="0"></c:set>
<c:forEach var="c" items="${sessionScope.cart}">
<c:set var="t" value="${t + c.producto.precio * c.cantidad}"></c:set>
</c:forEach>
<h4>Total Compra: ${t}</h4>
<form:form modelAttribute="orden" method="post" action="registrar" >
<div class="row">
<div class="input-field col s5">
<form:label path="nombre" >Nombre</form:label>
<form:input path="nombre" cssErrorClass="invalid input-error" />
<form:errors path="nombre" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="apellidoPaterno" >Apellido Paterno</form:label>
<form:input path="apellidoPaterno" cssErrorClass="invalid input-error" />
<form:errors path="apellidoPaterno" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="apellidoMaterno" >Apellido Materno</form:label>
<form:input path="apellidoMaterno" />
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="calle" >Calle y número</form:label>
<form:input path="calle" cssErrorClass="invalid input-error" />
<form:errors path="calle" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="entrecalles" >Entre que calles</form:label>
<form:input path="entrecalles" />
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="colonia" >Colonia</form:label>
<form:input path="colonia" cssErrorClass="invalid input-error" />
<form:errors path="colonia" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="municipio" >Municipio</form:label>
<form:input path="municipio" cssErrorClass="invalid input-error" />
<form:errors path="municipio" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="estado" >Estado</form:label>
<form:input path="estado" cssErrorClass="invalid input-error" />
<form:errors path="estado" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="pais" >País</form:label>
<form:input path="pais" cssErrorClass="invalid input-error" />
<form:errors path="pais" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="codigoPostal" >Código postal</form:label>
<form:input path="codigoPostal" cssErrorClass="invalid input-error" />
<form:errors path="codigoPostal" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="telefono" >Teléfono</form:label>
<form:input path="telefono" cssErrorClass="invalid input-error" />
<form:errors path="telefono" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col s5">
<form:label path="email" >Email</form:label>
<form:input path="email" cssErrorClass="invalid input-error" />
<form:errors path="email" cssClass="red-text text-error"/>
</div>
</div>
<div class="row">
<div class="input-field col">
<input type="submit" value="Registrar" class="btn waves-effect"/>
<a href="cancelarCompra" class="waves-effect waves-light btn">Cancelar Compra</a>
</div>
</div>
</form:form>
</div>
</body>
</html>
La ultima vista sería comprar.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link href="http://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link rel="stylesheet" type="text/css" href="css/style.css" />
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection"/>
<script type="text/javascript" src="https://code.jquery.com/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<title>Confirmar compra</title>
</head>
<body>
<div class="container">
<h1>Confirmar compra</h1>
<h2>Datos personales:</h2>
<table class="striped">
<thead>
</thead>
<tbody>
<tr>
<td> <span class="red-text text-darken-2">Nombre: </span> ${orden.nombre} ${orden.apellidoPaterno} ${orden.apellidoMaterno}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Calle y número: </span>${orden.calle}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Entrecalles: </span>${orden.entrecalles}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Colonia: </span>${orden.colonia}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Municipio: </span>${orden.municipio}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Estado: </span>${orden.estado}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">País: </span>${orden.pais}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Código Postal: </span>${orden.codigoPostal}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Teléfono: </span>${orden.telefono}</td>
</tr>
<tr>
<td><span class="red-text text-darken-2">Email: </span>${orden.email}</td>
</tr>
</tbody>
</table>
<h2>Orden:</h2>
<table class="bordered">
<thead>
<tr>
<th>Cantidad</th>
<th>Nombre Producto</th>
<th>Precio</th>
<th>Sub total</th>
</tr>
</thead>
<tbody>
<c:set var="t" value="0"></c:set>
<c:forEach var="c" items="${sessionScope.cart}">
<c:set var="t" value="${t + c.producto.precio * c.cantidad}"></c:set>
<tr>
<td>${c.cantidad}</td>
<td>${c.producto.nombreProducto}</td>
<td>${c.producto.precio}</td>
<td>${c.producto.precio * c.cantidad}</td>
</tr>
</c:forEach>
<tr>
<td colspan="3">Total</td>
<td>${t}</td>
</tr>
</tbody>
</table><br>
<form:form modelAttribute="orden" method="post" action="pagar">
<form:hidden path="nombre" value="${orden.nombre}"/>
<form:hidden path="apellidoPaterno" value="${orden.apellidoPaterno}"/>
<form:hidden path="apellidoMaterno" value="${orden.apellidoMaterno}"/>
<form:hidden path="calle" value="${orden.calle}"/>
<form:hidden path="entrecalles" value="${orden.entrecalles}"/>
<form:hidden path="colonia" value="${orden.colonia}"/>
<form:hidden path="municipio" value="${orden.municipio}"/>
<form:hidden path="estado" value="${orden.estado}"/>
<form:hidden path="pais" value="${orden.pais}"/>
<form:hidden path="codigoPostal" value="${orden.codigoPostal}"/>
<form:hidden path="telefono" value="${orden.telefono}"/>
<form:hidden path="email" value="${orden.email}"/>
<div class="row">
<div class="input-field col">
<input type="submit" value="Pagar" class="btn waves-effect"/>
<a href="cancelarCompra" class="waves-effect waves-light btn">Cancelar Compra</a>
</div>
</div>
</form:form>
</div>
</body>
</html>
En src/main/webapp creamos los folders css,js/fonts es donde van ir los archivos
de hojas estilo,javascript y tipo de letra aquín dejo un link para deecargar:
Una vez integrado todo comenzamos a testear el modulo corremos el proyecto:
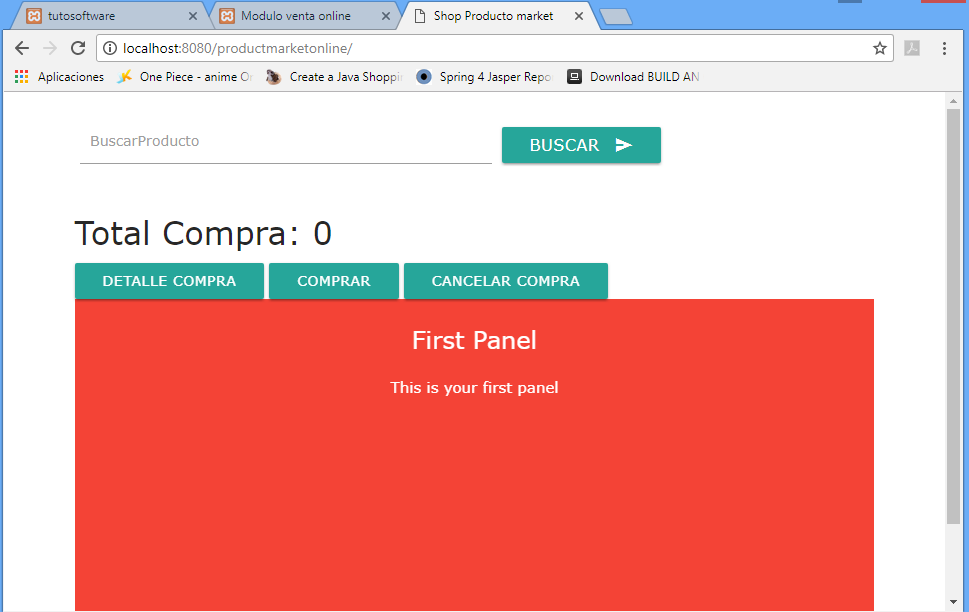
buscamos un producto y presionamos buscar:
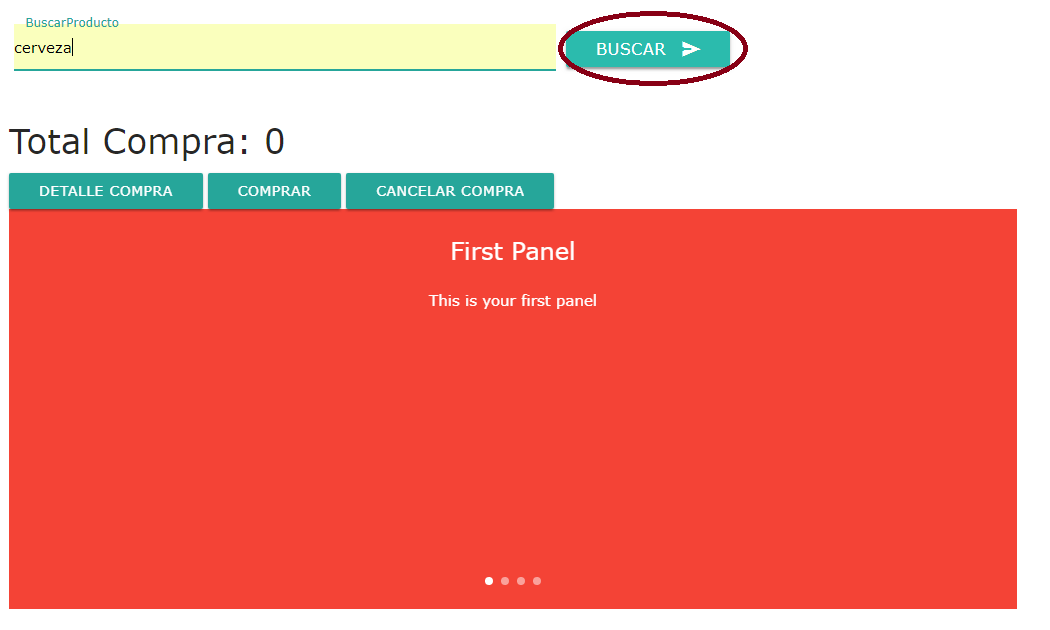
Esto es lo que nos arroja:
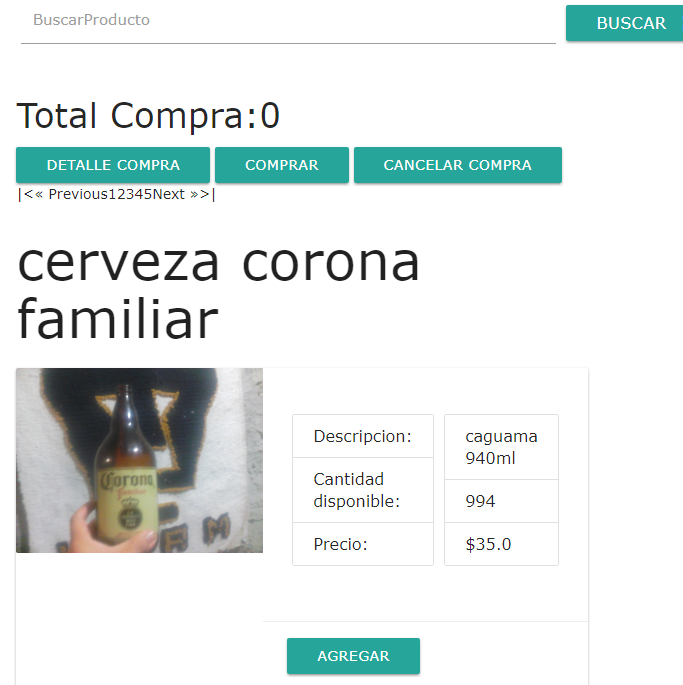
Ahora agregamos el producto y esto es lo que aparece:
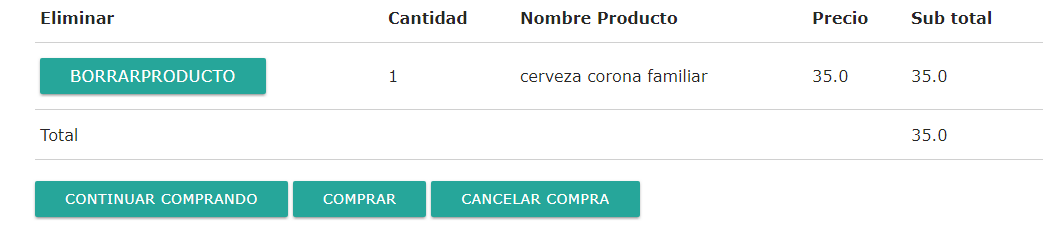
Seleccionamos continuar comprando buscamos otro producto y lo agregamos:
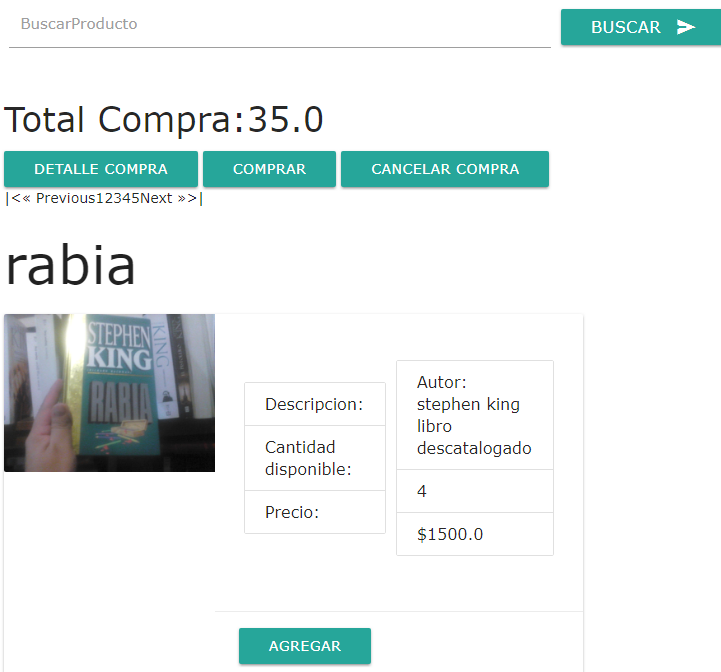
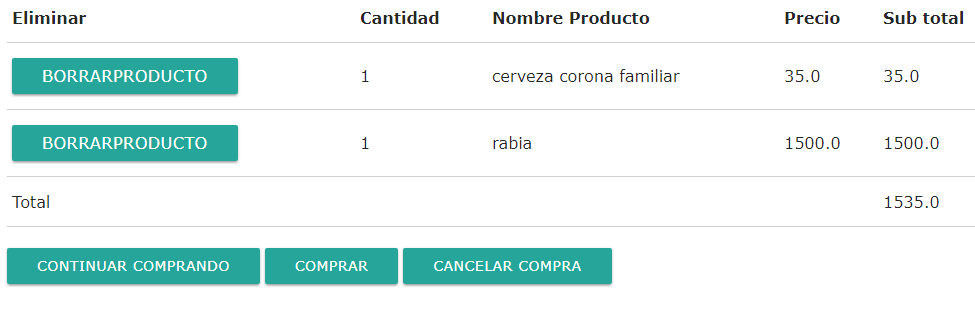
Ahora seleccionamos comprar
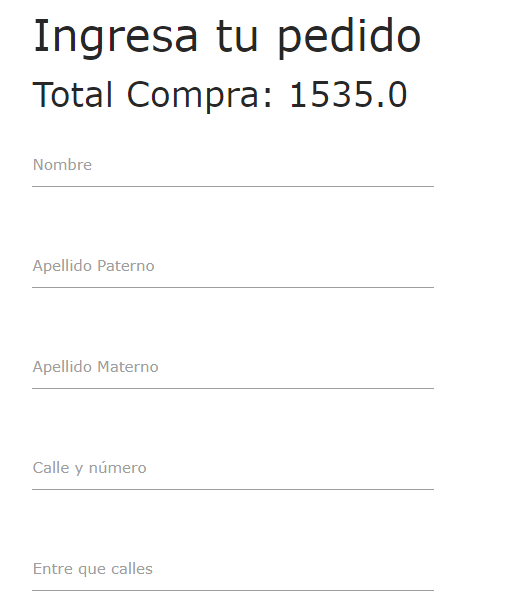
Llenamos nuestro pedido y le damos registrar
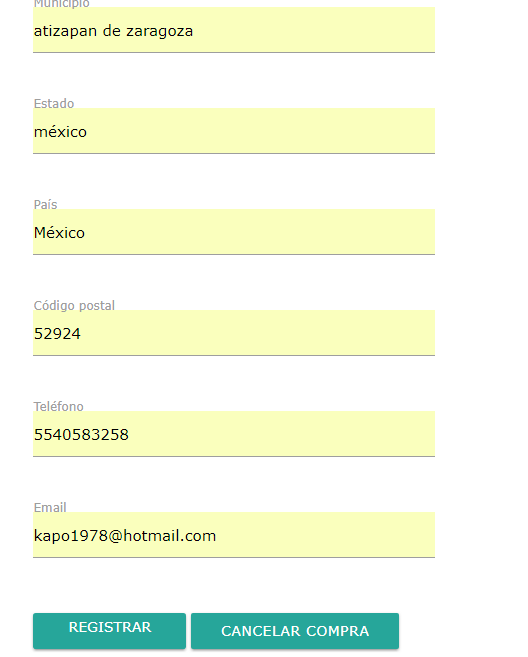
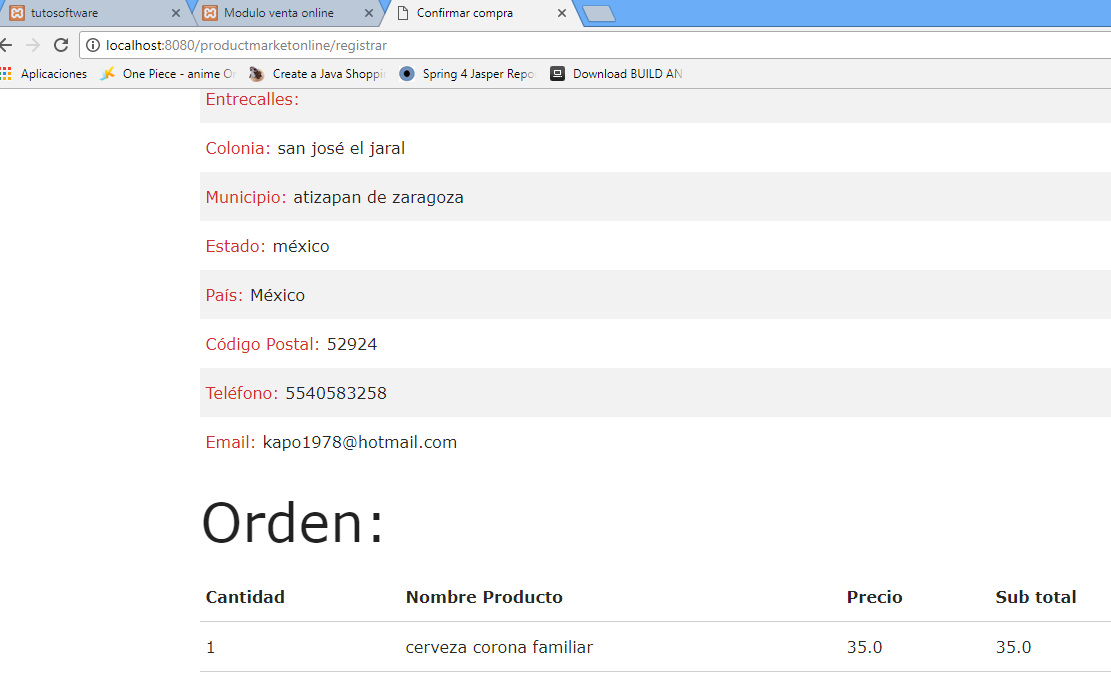
Ahora le damos en pagar:
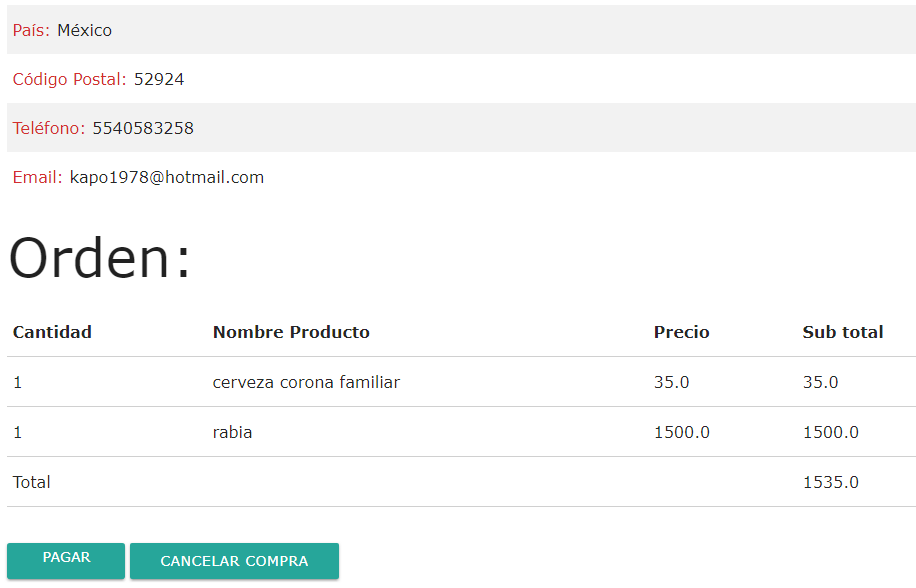
Listo realizamos nuestra compra,faltaron algunas validaciones y el tipo de
pago y la parte de envio pero esta fuera del alcance de este tutorial.Nada mas
ha sido para probar spring mvc ya que yo no lo habia trabajado.Pero ahora vamos por el modulo de
caja.