Punto de venta
Esta es la parte central o nucleo de nuestra aplicación aquí donde vamos a buscar nuestro productos mediante el código de barras ya sea tecleando el código de barras o con escaner con enter.Creamos un Windows Form y lo nombramos PuntoVenta.cs y lo nombramos frmPuntoVenta.
Agregamos un MenuStrip y encabeazado escribimos Opciones y las opciones las nombramos:
- Buscar Productos
- Menu
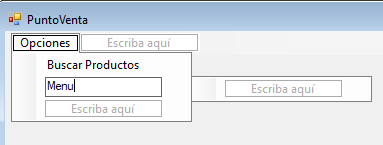
Agregamos un Label y en el texto ponemos Gato Abarrotero

Incluimos otro Label y en texto escribimos Código de Barras
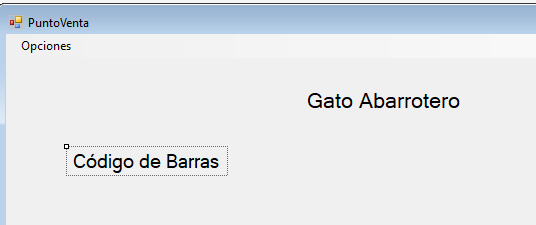
Seleccionamos un TextBox y lo nombramos txtCodigo
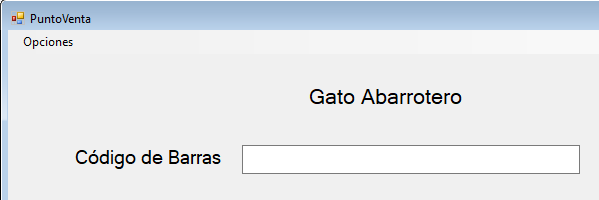
Añadimos un Button en el texto escribimos Agregar Producto y lo nombramos btnAgregarProducto

Agregamos un DataGridView y lo nombramos dtgvProductos

Seleccionamos Editar Columnas ...
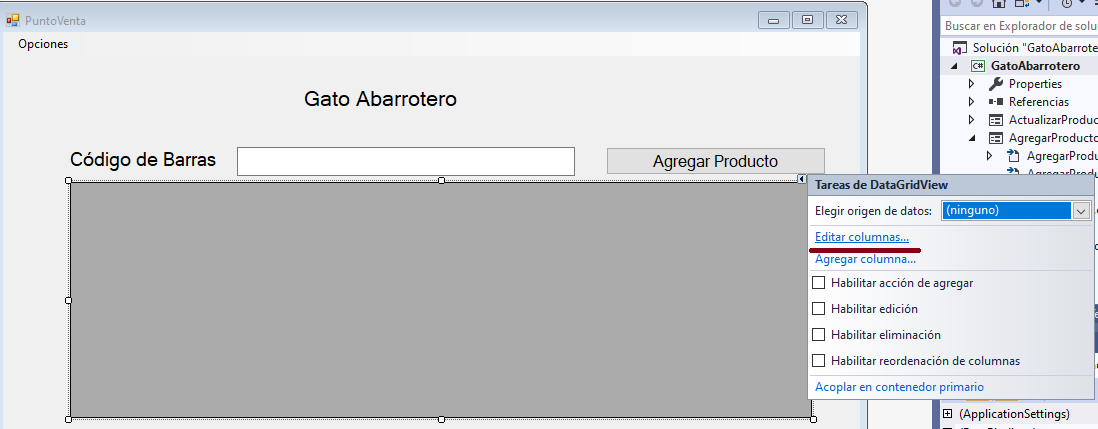
Le damos en agregar la primer columna la nombramos Cantidad y en el en el texto de encabezado ponemos Cantidad y le damos agregar.
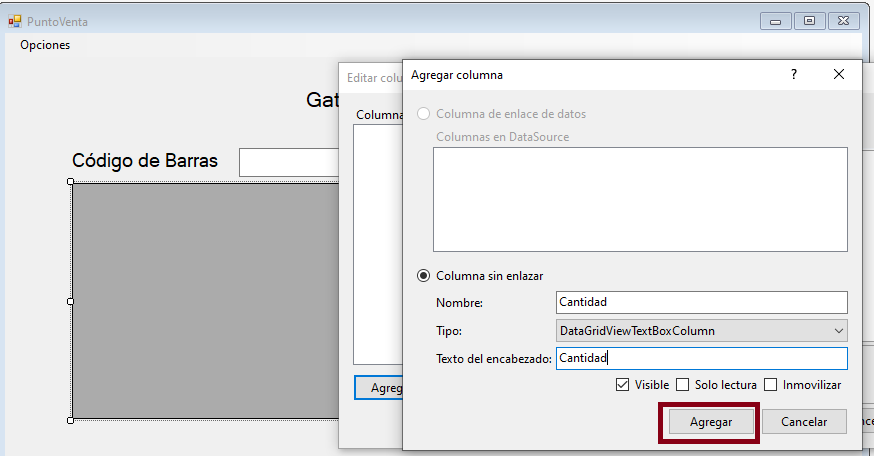
La siguiente Nombre:Codigo Texto de Encabezado Código y agregar

Siguiente Nombre:Producto Texto de Encabezado Nombre del Producto y agregar
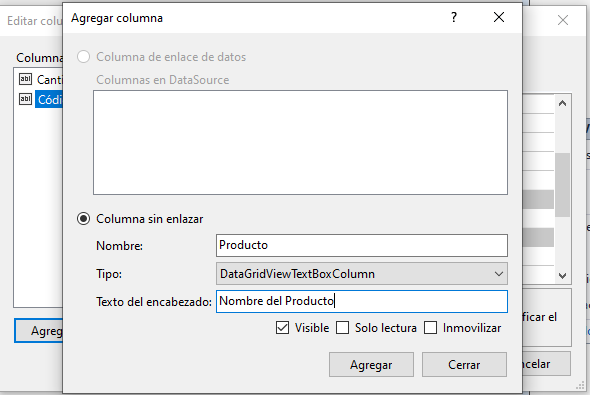
Siguiente Nombre:PrecioU Texto de Encabezado Precio Unitario y agregar
Posteriormente Cerrar
Luego le damos Aceptar
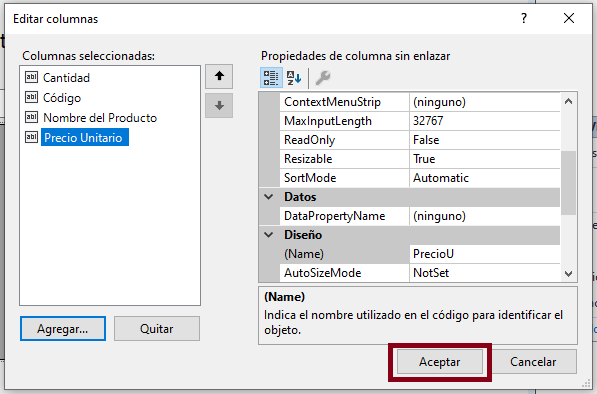

Copiamos y pegamos un Button en el texto ponemos Cancelar Compra y lo nombramos btnCancelarCompra

Copiamos y pegamos un Button en el texto ponemos Pagar y lo nombramos btnPagar

Ahora copiamos un Label y en el texto ponemos Total:
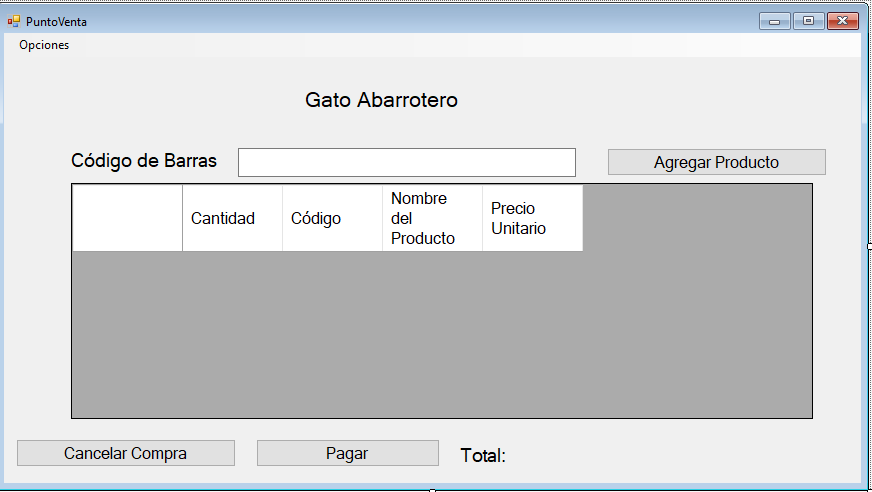
Para terminar el diseño copiamos y pegamos un TextBox y lo nombramos txtTotal
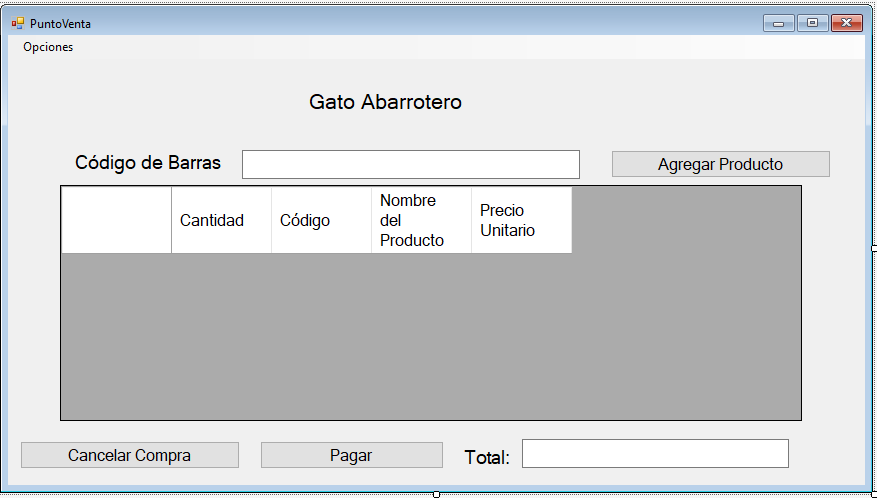
Nos vamos al código y inicalizamos nuestra conexión a base de datos.
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
}
}
}
A continuación creamos un clase llamada Producto.cs

Escribimos el siguiente código:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GatoAbarrotero
{
class Producto
{
public int cantidad { get; set; }
public string codigo { get; set; }
public string nombre { get; set; }
public decimal precio { get; set; }
}
}
Ahora el primer evento que vamos a colocar es la captura del código de barras con el escanner y el uso del enter.
using MySql.Data.MySqlClient; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace GatoAbarrotero { public partial class frmPuntoVenta : Form { MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb"); public frmPuntoVenta() { InitializeComponent(); InitializaeEvents(); } private void InitializaeEvents() { this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress); } private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e) { if (e.KeyChar == (char)Keys.Return) { try { conexion.Open(); string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' "; MySqlCommand cmd = new MySqlCommand(selectQuery, conexion); MySqlDataAdapter selecionnar = new MySqlDataAdapter(); selecionnar.SelectCommand = cmd; DataTable datosProducto = new DataTable(); selecionnar.Fill(datosProducto); if (datosProducto.Rows.Count == 0) { MessageBox.Show("No se encuenta el producto"); conexion.Close(); txtCodigo.Text = ""; } else { DataRow row = datosProducto.Rows[0]; Producto producto = new Producto(); producto.codigo = row["codigoBarras"].ToString(); producto.nombre = row["nombre"].ToString(); producto.precio = Convert.ToDecimal(row["precio"]); Boolean agregar = true; Boolean sumar = true; producto.cantidad = 1; decimal total = 0; if (dtgvProductos.Rows.Count > 0) { foreach (DataGridViewRow fila in dtgvProductos.Rows) { object valor = fila.Cells["Codigo"].Value; if (String.Compare(producto.codigo, valor.ToString()) == 0) { producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value); fila.Cells["Cantidad"].Value = producto.cantidad; total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad; agregar = false; } else { sumar = false; } } if (sumar == false) { total = Convert.ToDecimal(txtTotal.Text) + producto.precio; } if (agregar == true) { dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio); } txtTotal.Text = Convert.ToString(total); } else { dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio); total += producto.precio; txtTotal.Text = Convert.ToString(total); } conexion.Close(); } txtCodigo.Text = ""; } catch (MySqlException E) { MessageBox.Show(E.ToString()); } } } } }
En ocasiones el escanner no logra detectar el código de barras entonces capturamos el código y presionamos el Button agregar producto hacemos doble click en btnAgregarProducto CONSEJO: Aquí repeti código es impresindible evitar duplicación de código no tuve tiempo para generar un método, pero te lo dejo a tu criterio:
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
InitializaeEvents();
}
private void InitializaeEvents()
{
this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress);
}
private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Return)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
conexion.Close();
txtCodigo.Text = "";
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
}
txtCodigo.Text = "";
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
private void BtnAgregarProducto_Click(object sender, EventArgs e)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
txtCodigo.Text = "";
conexion.Close();
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
txtCodigo.Text = "";
}
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
}
Lo que sigue es habilitar el Button btnCancelarCompra para cuando cancelamos la compra:
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
InitializaeEvents();
}
private void InitializaeEvents()
{
this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress);
}
private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Return)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
conexion.Close();
txtCodigo.Text = "";
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
}
txtCodigo.Text = "";
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
private void BtnAgregarProducto_Click(object sender, EventArgs e)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
txtCodigo.Text = "";
conexion.Close();
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
txtCodigo.Text = "";
}
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
private void BtnCancelarCompra_Click(object sender, EventArgs e)
{
dtgvProductos.Rows.Clear();
dtgvProductos.Refresh();
}
}
}
Antes de realizar la función pagar vamos a crear un Windows Forms llamado Pagar.cs y lo nombramos frmPagar. Agregamos un Label y en texto escribimos Total:
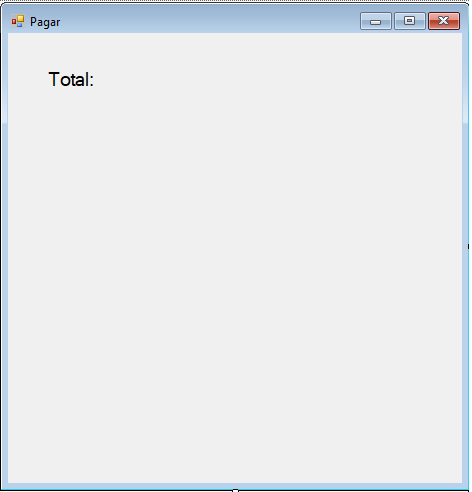
Seleccionamos un TextBox y lo nombramos txtTotal

El modificador lo ponemos como public porque el valor total lo vamos a mandar de un formulario a otro

Copiamos y pegamos el Label y en el texto ponemos Efectivo:

Copiamos y pegamos el TextBox y lo nombramos txtEfectivo

Copiamos y pegamos el Label y en el texto ponemos Cambio:
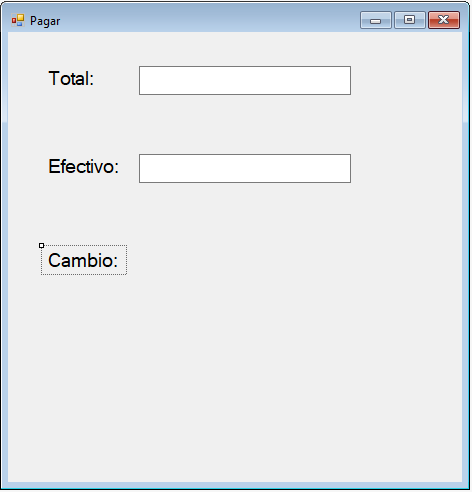
Copiamos y pegamos el TextBox y lo nombramos txtCambio

Agregamos un Button y en el texto escribimos Cobrar y lo nombramos btnCobrar

Copiamos y pegamos el Button en el texto ponemos Cerrar y lo nombramos btnCerrar

Volvemos a Puntoventa.cs[diseño] y hacemos doble click en btnPagar
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
InitializaeEvents();
}
private void InitializaeEvents()
{
this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress);
}
private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Return)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
conexion.Close();
txtCodigo.Text = "";
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
}
txtCodigo.Text = "";
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
private void BtnAgregarProducto_Click(object sender, EventArgs e)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
txtCodigo.Text = "";
conexion.Close();
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
txtCodigo.Text = "";
}
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
private void BtnCancelarCompra_Click(object sender, EventArgs e)
{
dtgvProductos.Rows.Clear();
dtgvProductos.Refresh();
}
private void BtnPagar_Click(object sender, EventArgs e)
{
DataTable data = new DataTable();
foreach (DataGridViewColumn columna in this.dtgvProductos.Columns)
{
DataColumn col = new DataColumn(columna.Name);
data.Columns.Add(col);
}
//Recorrer filas
foreach (DataGridViewRow fila in this.dtgvProductos.Rows)
{
DataRow dr = data.NewRow();
dr[0] = fila.Cells[0].Value.ToString();
dr[1] = fila.Cells[1].Value.ToString();
dr[2] = fila.Cells[2].Value.ToString();
dr[3] = fila.Cells[3].Value.ToString();
data.Rows.Add(dr);
}
dtgvProductos.DataSource = data;
frmPagar pg = new frmPagar(data);//En el otro código se crea el contructor
pg.txtTotal.Text = txtTotal.Text;
pg.Show();
this.Hide();
}
}
}
Nos vamos al menú opciones y damos doble click en la opción Buscar Productos
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
InitializaeEvents();
}
private void InitializaeEvents()
{
this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress);
}
private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Return)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
conexion.Close();
txtCodigo.Text = "";
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
}
txtCodigo.Text = "";
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
private void BtnAgregarProducto_Click(object sender, EventArgs e)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
txtCodigo.Text = "";
conexion.Close();
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
txtCodigo.Text = "";
}
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
private void BtnCancelarCompra_Click(object sender, EventArgs e)
{
dtgvProductos.Rows.Clear();
dtgvProductos.Refresh();
}
private void BtnPagar_Click(object sender, EventArgs e)
{
DataTable data = new DataTable();
foreach (DataGridViewColumn columna in this.dtgvProductos.Columns)
{
DataColumn col = new DataColumn(columna.Name);
data.Columns.Add(col);
}
//Recorrer filas
foreach (DataGridViewRow fila in this.dtgvProductos.Rows)
{
DataRow dr = data.NewRow();
dr[0] = fila.Cells[0].Value.ToString();
dr[1] = fila.Cells[1].Value.ToString();
dr[2] = fila.Cells[2].Value.ToString();
dr[3] = fila.Cells[3].Value.ToString();
data.Rows.Add(dr);
}
dtgvProductos.DataSource = data;
frmPagar pg = new frmPagar(data);//En el otro código se crea el contructor
pg.txtTotal.Text = txtTotal.Text;
pg.Show();
this.Hide();
}
private void BuscarProductosToolStripMenuItem_Click(object sender, EventArgs e)
{
frmVerProducto buscar = new frmVerProducto();
buscar.Show();
}
}
}
Por último seleccionamos de menú opciones la opcion Menú y hacemos doble click
using MySql.Data.MySqlClient;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace GatoAbarrotero
{
public partial class frmPuntoVenta : Form
{
MySqlConnection conexion = new MySqlConnection("server=localhost;User id=admin;password=adminlara;database=puntodeventadb");
public frmPuntoVenta()
{
InitializeComponent();
InitializaeEvents();
}
private void InitializaeEvents()
{
this.txtCodigo.KeyPress += new KeyPressEventHandler(TxtCodigo_KeyPress);
}
private void TxtCodigo_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Return)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
conexion.Close();
txtCodigo.Text = "";
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
}
txtCodigo.Text = "";
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
}
private void BtnAgregarProducto_Click(object sender, EventArgs e)
{
try
{
conexion.Open();
string selectQuery = "SELECT codigoBarras,nombre,precio FROM producto WHERE codigoBarras ='" + txtCodigo.Text + "' ";
MySqlCommand cmd = new MySqlCommand(selectQuery, conexion);
MySqlDataAdapter selecionnar = new MySqlDataAdapter();
selecionnar.SelectCommand = cmd;
DataTable datosProducto = new DataTable();
selecionnar.Fill(datosProducto);
if (datosProducto.Rows.Count == 0)
{
MessageBox.Show("No se encuenta el producto");
txtCodigo.Text = "";
conexion.Close();
}
else
{
DataRow row = datosProducto.Rows[0];
Producto producto = new Producto();
producto.codigo = row["codigoBarras"].ToString();
producto.nombre = row["nombre"].ToString();
producto.precio = Convert.ToDecimal(row["precio"]);
Boolean agregar = true;
Boolean sumar = true;
producto.cantidad = 1;
decimal total = 0;
if (dtgvProductos.Rows.Count > 0)
{
foreach (DataGridViewRow fila in dtgvProductos.Rows)
{
object valor = fila.Cells["Codigo"].Value;
if (String.Compare(producto.codigo, valor.ToString()) == 0)
{
producto.cantidad += Convert.ToInt32(fila.Cells["Cantidad"].Value);
fila.Cells["Cantidad"].Value = producto.cantidad;
total += Convert.ToDecimal(fila.Cells["PrecioU"].Value) * producto.cantidad;
agregar = false;
}
else
{
sumar = false;
}
}
if (sumar == false)
{
total = Convert.ToDecimal(txtTotal.Text) + producto.precio;
}
if (agregar == true)
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
}
txtTotal.Text = Convert.ToString(total);
}
else
{
dtgvProductos.Rows.Add(producto.cantidad, producto.codigo, producto.nombre, producto.precio);
total += producto.precio;
txtTotal.Text = Convert.ToString(total);
}
conexion.Close();
txtCodigo.Text = "";
}
}
catch (MySqlException E)
{
MessageBox.Show(E.ToString());
}
}
private void BtnCancelarCompra_Click(object sender, EventArgs e)
{
dtgvProductos.Rows.Clear();
dtgvProductos.Refresh();
}
private void BtnPagar_Click(object sender, EventArgs e)
{
DataTable data = new DataTable();
foreach (DataGridViewColumn columna in this.dtgvProductos.Columns)
{
DataColumn col = new DataColumn(columna.Name);
data.Columns.Add(col);
}
//Recorrer filas
foreach (DataGridViewRow fila in this.dtgvProductos.Rows)
{
DataRow dr = data.NewRow();
dr[0] = fila.Cells[0].Value.ToString();
dr[1] = fila.Cells[1].Value.ToString();
dr[2] = fila.Cells[2].Value.ToString();
dr[3] = fila.Cells[3].Value.ToString();
data.Rows.Add(dr);
}
dtgvProductos.DataSource = data;
frmPagar pg = new frmPagar(data);//En el otro código se crea el contructor
pg.txtTotal.Text = txtTotal.Text;
pg.Show();
this.Hide();
}
private void BuscarProductosToolStripMenuItem_Click(object sender, EventArgs e)
{
frmVerProducto buscar = new frmVerProducto();
buscar.Show();
}
private void MenuToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Hide();
frmMenu menu = new frmMenu();
menu.Show();
}
}
}